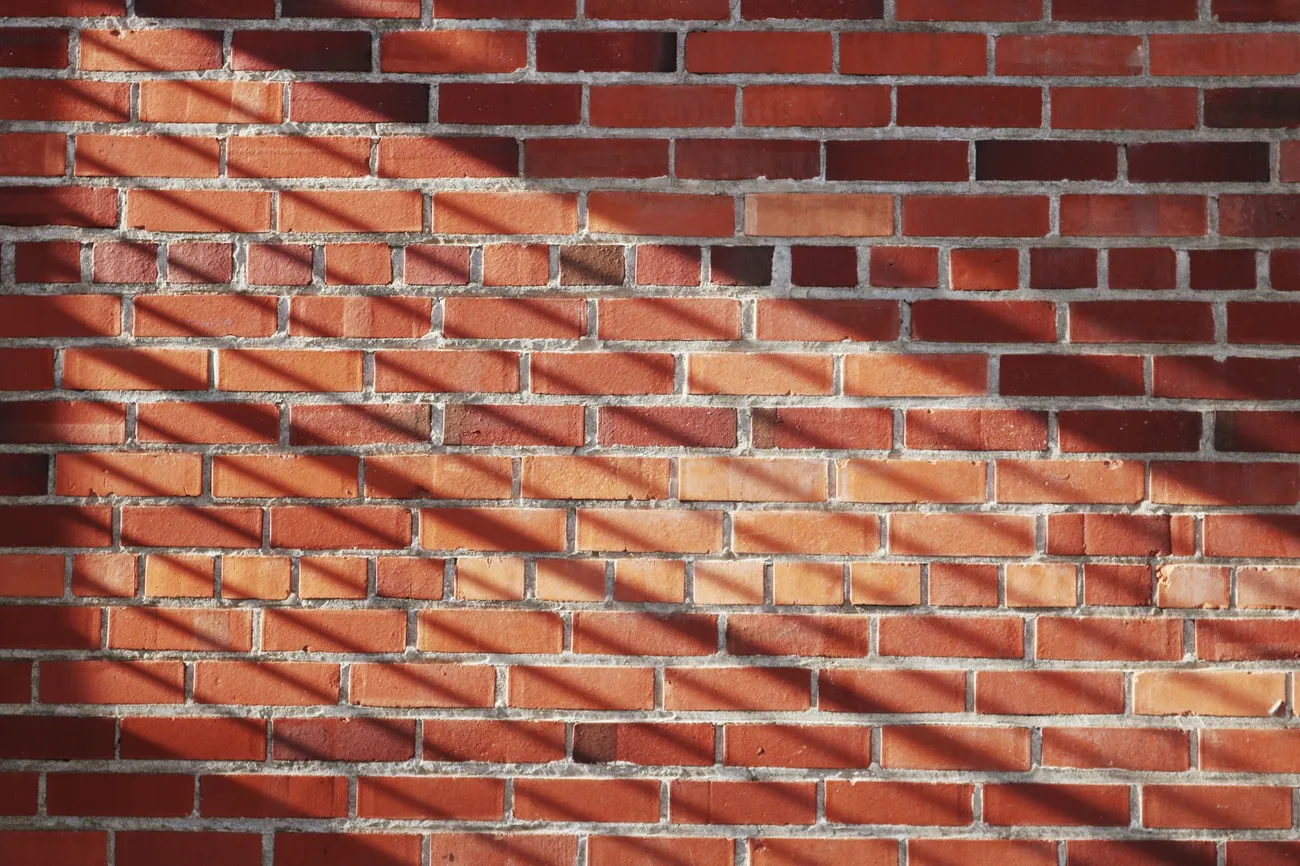
Masonry layout for Vue 2 and Vue 3
Responsive masonry layout with SSR support and zero dependencies for Vue 2 and Vue 3.
IntroductionSection titled Introduction
In quite a few of my Vue 2 projects I’m using the library vue-masonry-wall. Its ease-of-use and SSR support were key factors in my decision to use this library in particular.
However, I’m currently in the progress of preparing my projects for the release of Nuxt 3 and the accompanying migration to Vue 3.
Since I couldn’t find a comparable masonry layout for Vue 3, I chose to create a new library based on Fuxing Loh’s vue-masonry-wall
.
The result is @yeger/vue-masonry-wall, a rewrite of the original library in TypeScript and Vue 3. It has no dependencies, resulting in a bundle size decrease of up to 54%.
I also created @yeger/vue2-masonry-wall for use in Vue 2 projects. It, too, drops any dependencies, achieving a bundle size decrease of up to 40%
In addition, multiple issues have been fixed.
Notably, spacing (gap
) is now considered while calculating the column count, and the removal of elements is now supported.
InstallationSection titled Installation
Vue 2Section titled Vue 2
# yarn
$ yarn add @yeger/vue2-masonry-wall
# npm
$ npm install @yeger/vue2-masonry-wall
First, you have to install the component like any other plugin. Consult the Vue 2 documentation for detailed information on installing plugins.
import MasonryWall from '@yeger/vue2-masonry-wall'
import Vue from 'vue'
Vue.use(MasonryWall)
Vue 3Section titled Vue 3
# yarn
$ yarn add @yeger/vue-masonry-wall
# npm
$ npm install @yeger/vue-masonry-wall
First, you have to install the component like any other plugin. Consult the Vue 3 documentation for detailed information on installing plugins.
import MasonryWall from '@yeger/vue-masonry-wall'
import { createApp } from 'vue'
const app = createApp()
app.use(MasonryWall)
UsageSection titled Usage
The component is available as masonry-wall
or MasonryWall
.
The only required prop is items
, an array of type any
.
Each element of items
is passed to the default slot, alongside its index.
The prop column-width
takes in a number
, specifying the targeted column width.
It is used to calculate the column count but does not define the actual width of columns.
Similarly, gap
defines the gaps between items in pixels and is respected while calculating the column count.
Lastly, the prop ssr-columns
can be used to specify the column count in server-side-rendering contexts.
While every prop of the component is reactive, mutations to the items
array will not update the layout.
To force updates, assign a new array to the items
prop.
ExampleSection titled Example
This example demonstrates basic usage of the component. For interactive demonstrations, visit vue-masonry-wall.yeger.eu or vue2-masonry-wall.yeger.eu.
<script lang="ts">
import MasonryWall from '@yeger/vue-masonry-wall'
import { defineComponent } from 'vue'
export default defineComponent({
components: {
MasonryWall,
},
data() {
return {
items: [50, 75, 75, 100, 50, 50, 75, 150, 125, 175, 50, 100, 125],
}
},
})
</script>
<template>
<MasonryWall :items="items" :ssr-columns="1" :column-width="100" :gap="16">
<template #default="{ item, index }">
<div
:style="{ height: `${item}px` }"
class="card flex items-center justify-center"
>
{{ index }}
</div>
</template>
</MasonryWall>
</template>
The above code results in the following output.